Programming Made Simple!
Bringing an easy to learn and use language to the mobile world and the Android platform is the goal of the Simple project. Simple is a BASIC dialect for developing Android applications. It is particularly well suited for non-professional programmers (but not limited to). Simple allows programmers to quickly write Android applications by using the components supplied by its runtime system.
Similar to its 90s relative, Simple programs are form definitions (which contain components) and code (which contains the program logic). The interaction between the components and the program logic happens through events triggered by the components. The program logic consists of event handlers which contain code reacting to the events. In reality it is even simpler than this description.
Let's see how simple it really is. We will quickly write a program simulating the famous Etch-A-Sketch on an Android device. Tilting the device will move the pen, shaking the device will clear the screen. The Simple runtime system gives us three components to provide most of the needed functionality:
- the Canvas component - for drawing
- the OrientationSensor component - to detect tilting
- the Accelerometer component to detect shaking
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | Dim x As Integer Dim y As Integer Event OrientationSensor1.OrientationChanged(yaw As Single, _ pitch As Single, roll As Single) If roll < -20 Then x = Math.Min(Canvas1.Width, x + 1) ElseIf roll > 20 Then x = Math.Max(0, x - 1) End If If pitch < -20 Then y = Math.Min(Canvas1.Height, y + 1) ElseIf pitch > 20 Then y = Math.Max(0, y - 1) End If Canvas1.DrawPoint(x, y) End Event Event AccelerometerSensor1.Shaking() Canvas1.Clear() End Event |
The code defines two global variables (lines 1 and 2) and two event handlers, one to handle changes in the device's tilt (lines 4 to 17) and another to handle shaking of the device (lines 19 to 21). The code in the firstevent handler makes sure to only react to tilting above a certain degree (lines 6, 8, 11 and 13), and if that is the case then it further ensures that the pen does not run off the drawing surface (lines 7, 9, 12 and 14). And finally a point is drawn at the pen position (line 16). As for the other event handler, the only thing it does is clearing the drawing surface in case of shaking (line 20).
Last part missing is the form definition. It defines the form and its properties (lines 24 to 27), followed by the components it contains (lines 28 to 33).
22 23 24 25 26 27 28 29 30 31 32 33 34 35 | $Properties $Source $Form $Define EtchSketch $As Form Layout = 3 BackgroundColor = &HFFFFFFFF Title = "EtchSketch: Tilt to draw - Shake to clear" $Define Canvas1 $As Canvas $End $Define $Define OrientationSensor1 $As OrientationSensor $End $Define $Define AccelerometerSensor1 $As AccelerometerSensor $End $Define $End $Define $End $Properties |
That's it. The only thing left to do is to compile and deploy the application to an Android device. And voila, here is a screenshot of the application running:
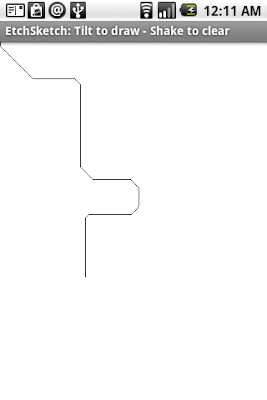
Programming made Simple!
(This is a copy of my original post on the 'Open Source at Google' blog)